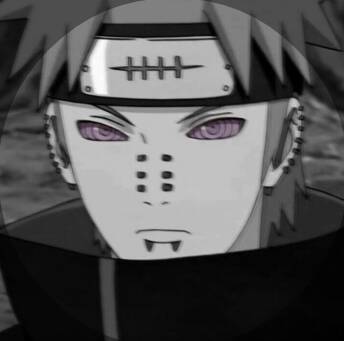
Laucher
2023年 08月 24日
JavaScript 数组常用方法
本文将针对JavaScript,详细介绍一系列常用的数组方法,涵盖了flatMap()、concat()、copyWithin()、every()、fill()、filter()、flat()、forEach()、shift()、includes() 以及 reduce()。通过掌握这些方法,您可以在实际开发中提高工作效率并改善代码质量。
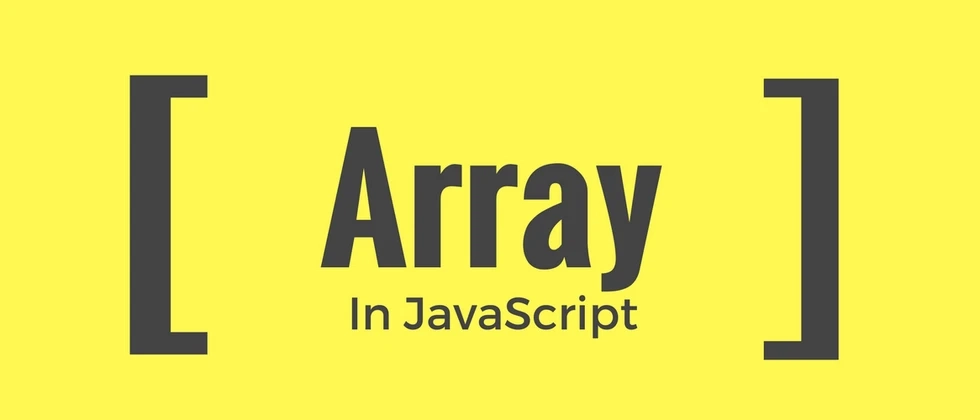
在JavaScript中,数组是一种常见且必不可少的数据结构,用于存储一系列值。数组方法是对数组进行操作和转换的强大工具。本文将详细解释一些常用的数组方法,包括concat()、copyWithin()、every()、fill()、filter()、flat()、forEach()、shift()、includes()、flatMap() 以及 reduce()。
1. concat()
concat()方法是用于合并两个或多个数组,特别是在需要合并多个数据源或动态生成数组时。它不会修改原始数组,而是返回一个新的数组,其中包含合并的元素。
const array1 = ['猴子', '猿', '大猩猩'];
const array2 = ['山羊', '绵羊', '公羊'];
const newArray = array1.concat(array2);
console.log(newArray); // 输出: ['猴子', '猿', '大猩猩', '山羊', '绵羊', '公羊']
2. copyWithin()
copyWithin()方法允许我们在数组内部复制相同的数组元素,从而修改原始数组并返回对修改后数组的引用。它接受三个参数:目标位置、源起始位置和源结束位置。
const numArray = [1, 2, 3, 4, 5];
numArray.copyWithin(0, 3, 5);
console.log(numArray); // 输出: [4, 5, 3, 4, 5]
同时,copyWithin()方法在需要在数组内部重新排列元素时非常有用,例如在实现排序算法时。
const unsortedArray = [3, 1, 4, 1, 5, 9, 2, 6];
// 将最小的三个元素移到数组开头
unsortedArray.copyWithin(0, unsortedArray.indexOf(1), unsortedArray.indexOf(1) + 3);
// unsortedArray: [1, 5, 9, 1, 5, 9, 2, 6]
3. every()
every()方法对数组的每个元素应用一个测试函数(验证数组中的所有元素是否满足某个条件),如果所有元素都通过测试(满足条件),则返回true
,否则返回false
。
const numArray = [2, 4, 6, 8];
const allEven = numArray.every(num => num % 2 === 0);
console.log(allEven); // 输出: true
const scores = [85, 90, 78, 95, 88];
const allPassed = scores.every(score => score >= 80);
console.log(allPassed); // 输出: false
4. fill()
fill()方法用指定的静态值填充数组中的一段区间,不会改变原始数组,而是返回一个新的数组。
const strArray = ['python', 'java', 'golang', 'ruby', 'reactjs'];
strArray.fill('css', 1, 3);
console.log(strArray); // 输出: ['python', 'css', 'css', 'ruby', 'reactjs']
同时,fill()方法在需要创建具有特定大小和初始值的数组时非常有用,例如在图像处理中创建像素数组。
const pixelData = new Array(100).fill(0);
// pixelData: [0, 0, 0, ..., 0] (共有100个0)
5. filter()
filter()方法创建一个新数组,其中包含通过指定测试函数的所有元素。它不会改变原始数组。
const itemsArray = ['乐队', '鞋', '裙子', '包'];
const filteredArray = itemsArray.filter(str => str.startsWith('鞋'));
console.log(filteredArray); // 输出: ['鞋']
例如在搜索和过滤功能中使用。
const products = [
{ name: '苹果', category: '水果' },
{ name: '胡萝卜', category: '蔬菜' },
{ name: '香蕉', category: '水果' },
// ...
];
const fruits = products.filter(product => product.category === '水果');
// fruits: [{ name: '苹果', category: '水果' }, { name: '香蕉', category: '水果' }]
const vegetables = products.filter(product => product.category === '蔬菜');
// vegetables: [{ name: '胡萝卜', category: '蔬菜' }]
6. flat()
flat()方法可用于将嵌套的数组“拉平”,创建一个新的一维数组。它接受一个可选参数,指定展开的深度。
const nestedArray = ["你好", ["世界", "!"]];
const flattened = nestedArray.flat();
console.log(flattened); // flattened: ["你好", "世界", "!"]
并且flat()方法在处理嵌套的不规则数组结构时很有用,例如在API响应数据中处理嵌套的JSON对象。
const nestedData = [1, [2, [3, 4]], 5, [6]];
const flattenedData = nestedData.flat(2);
console.log(flattenedData); // flattenedData: [1, 2, 3, 4, 5, 6]
7. forEach()
forEach()方法遍历数组的每个元素,并对每个元素执行一个提供的函数。
const animalArray = ['山羊', '绵羊', '公羊'];
animalArray.forEach(animal => console.log(animal));
// 输出:
// 山羊
// 绵羊
// 公羊
同时forEach()方法通常用于迭代数组并执行某些副作用,例如在DOM操作中更新元素。
const elements = document.querySelectorAll('.item');
elements.forEach(element => {
element.style.backgroundColor = 'blue';
});
8. shift()
shift()方法用于从数组的头部删除第一个元素,并返回被删除的元素。这会修改原始数组。
const numArray = [1, 2, 3, 4, 5];
const firstElement = numArray.shift();
console.log(firstElement); // 输出: 1
console.log(numArray); // 输出: [2, 3, 4, 5]
9. flatMap()
flatMap()方法首先对数组中的每个元素执行提供的函数,然后将所有结果组成一个新数组。与map()不同的是,flatMap()会将嵌套数组的结果“扁平化”。
const array = [1, 2, 3, 4];
const flattenedArray = array.flatMap(num => [num, num * 2]);
// flattenedArray: [1, 2, 2, 4, 3, 6, 4, 8]
同时,flatMap()方法在处理嵌套数组时非常有用,特别是在需要展平数组并对每个元素执行操作的情况下。
const array = [
[1, 2, 3],
[4, 5],
[6, 7, 8]
];
const squaredAndFlattened = array.flatMap(innerArray => innerArray.map(num => num * num));
// squaredAndFlattened: [1, 4, 9, 16, 25, 36, 49, 64]
10. includes()
includes()方法用于在数组中查找特定元素是否存在,返回布尔值。例如在关键字搜索功能中判断关键字是否出现在文本中。
const keywords = ['JavaScript', 'array', 'methods'];
const hasKeyword = keywords.includes('array');
// hasKeyword: true
11. reduce()
reduce()方法在需要将数组元素合并为单个值时非常有用,它接受一个函数作为参数来执行计算。例如在计算总和、平均值或复杂的聚合操作。
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((total, num) => total + num, 0);
// sum: 15
这些详细解释的数组方法将帮助您更好地理解它们的功能和用法。通过灵活使用这些方法,您可以高效地处理和操作数组数据来解决实际问题。同时还为每个方法链接了一个MDN链接,它提供更佳全面的解释、示例和用法说明,如果您想更详细学习,请点击前往。